- 5. Combine multiple tests in a group
- 6. Run the tests
Some cross-platform (Android and iOS shared code base) mobile AR poc, by embedding an AR Foundation @unity3d scene in @FlutterDev. You can use this to add an AR feature to your existing #flutter app! Pic.twitter.com/hQEzFiWQYM — Thomas Stockx (@thomasstockx) August 21, 2019 12 comments. Flutter / Unity. 2020/06/07 Mathru/Kanimiso-P's Vocaloid music is made into free! 2020/05/12 Unity UIElements Expansions: Template.
How can you ensure that your app continues to work as youadd more features or change existing functionality?By writing tests.
Unit tests are handy for verifying the behavior of a single function,method, or class. The test
package provides thecore framework for writing unit tests, and the flutter_test
package provides additional utilities for testing widgets.
This recipe demonstrates the core features provided by the test
packageusing the following steps:
- Add the
test
orflutter_test
dependency. - Create a test file.
- Create a class to test.
- Write a
test
for our class. - Combine multiple tests in a
group
. - Run the tests.
For more information about the test package,see the test package documentation.
1. Add the test dependency
The test
package provides the core functionality for writing tests in Dart. This is the best approach whenwriting packages consumed by web, server, and Flutter apps.
2. Create a test file
In this example, create two files: counter.dart
and counter_test.dart
.
The counter.dart
file contains a class that you want to test andresides in the lib
folder. The counter_test.dart
file containsthe tests themselves and lives inside the test
folder.
In general, test files should reside inside a test
folderlocated at the root of your Flutter application or package.Test files should always end with _test.dart
,this is the convention used by the test runner when searching for tests.
When you’re finished, the folder structure should look like this:
3. Create a class to test
Next, you need a “unit” to test. Remember: “unit” is another name for afunction, method, or class. For this example, create a Counter
classinside the lib/counter.dart
file. It is responsible for incrementingand decrementing a value
starting at 0
.
Note: For simplicity, this tutorial does not follow the “Test DrivenDevelopment” approach. If you’re more comfortable with that style ofdevelopment, you can always go that route.
4. Write a test for our class
Inside the counter_test.dart
file, write the first unit test. Tests aredefined using the top-level test
function, and you can check if the resultsare correct by using the top-level expect
function.Both of these functions come from the test
package.
5. Combine multiple tests in a group
If you have several tests that are related to one another,combine them using the group
function provided by the test
package.
6. Run the tests
Now that you have a Counter
class with tests in place,you can run the tests.
Run tests using IntelliJ or VSCode
The Flutter plugins for IntelliJ and VSCode support running tests.This is often the best option while writing tests because it provides thefastest feedback loop as well as the ability to set breakpoints.
- IntelliJ
- Open the
counter_test.dart
file - Select the
Run
menu - Click the
Run 'tests in counter_test.dart'
option - Alternatively, use the appropriate keyboard shortcutfor your platform.
- Open the
- VSCode
- Open the
counter_test.dart
file - Select the
Run
menu - Click the
Start Debugging
option - Alternatively, use the appropriate keyboard shortcutfor your platform.
- Open the
Run tests in a terminal
You can also use a terminal to run the tests by executing the followingcommand from the root of the project:
For more options regarding unit tests, you can execute this command:
Flutter unity 3D widget for embedding unity in flutter. Now you can make awesome gamified features of your app in Unity and get it rendered in a Flutter app both in fullscreen and embeddable mode. Works great on Android, iPad OS and iOS. There are now two unity app examples in the unity folder, one with the default scene and another based on Unity AR foundation samples.
Note: Supports only Unity 2019.4.3 or later. UnityFramework does not support emulator.
Installation #
First depend on the library by adding this to your packages pubspec.yaml
:
Null-safe version:
Now inside your Dart code you can import it.

Preview #
30 fps gifs, showcasing communication between Flutter and Unity:
Setup #
For this, there is also a video tutorial, which you can find a here.
In the tutorial below, there are steps specific to each platform, denoted by a ℹ️ icon followed bythe platform name (Android or iOS). You can click on its icon to expand it.
Prerequisites #
An existing Flutter project (if there is none, you can create a new one)
An existing Unity project (if there is none, you can create a new one).
A
FlutterUnityPackage.unitypackage
file (you can access the Unity packages in the scripts folder too)
Steps #
- Create a folder named unity and move the Unity project into there.
The expected path is unity/project-name/...
- Copy the FlutterUnityPackage.unitypackage file into the Unity project folder.
The expected path is unity/project-name/FlutterUnityPackage.unitypackage
- Using Unity, open the Unity project, go to File > Build Settings > Player Settings and change the following under the Configuration section:
In Scripting Backend, change to IL2CPP
In Target Architectures, select ARMv7 and ARM64
Select the appropriate SDK on Target SDK depending on where you want to test or run your app (simulator or physical device).
Be sure you have at least one scene added to your build.
Go to Assets > Import Package > Custom Package and select the FlutterUnityPackage.unitypackage file. Click on Import.
After importing, click on Flutter and select the Export Android option (will export to android/unityLibrary) or the Export iOS option (will export to ios/UnityLibrary).
Do not use Flutter > Export Platform plugin as it was specially added to work with flutter_unity_cli
for larger projects.
The export script automatically sets things up for you, so you don't have to do anything for Android. But if you want to manually set it up, continue.
6.1. Open the android/settings.gradle file and change the following:
6.2. Open the android/app/build.gradle file and change the following:
6.3. If you need to build a release package, open the android/app/build.gradle file and change the following:
The code above use the debug
signConfig for all buildTypes, which can be changed as you well if you need specify signConfig.
6.4. If you use minifyEnabled true
in your android/app/build.gradle file, open the android/unityLibrary/proguard-unity.txt and change the following:
6.5. If you want Unity in it's own activity as an alternative, open the android/app/src/main/AndroidManifest.xml and change the following:
6.1. Open the ios/Runner.xcworkspace (workspace, not the project) file in Xcode, right-click on the Navigator (not on an item), go to Add Files to 'Runner' and add the ios/UnityLibrary/Unity-Iphone.xcodeproj file.
6.2. (Optional) Select the Unity-iPhone/Data folder and change the Target Membership for Data folder to UnityFramework.
6.3.1. If you're using Swift, open the ios/Runner/AppDelegate.swift file and change the following:
6.3.2. If you're using Objective-C, open the ios/Runner/main.m file and change the following:
6.4. Open the ios/Runner/Info.plist and change the following:
6.5. Add the UnityFramework.framework file as a library to the Runner project.
Setup AR Foundation
Check out the Unity AR Foundation samples in the demo repository.This repository is not guaranteed to be up-to-date with the latest flutter-unity-view-widget
master. Make sure to followthe steps listed below for setting up AR Foundation on iOS and Android in your project.
Open the lib/architecture/ folder and check if there are both libUnityARCore.so and libarpresto_api.so files. There seems to be a bug where a Unity export does not include all lib files. If they are missing, use Unity to build a standalone .apk of your AR project, unzip the resulting apk, and copy over the missing .lib files to the
unityLibrary
module.Repeat steps 6.1 and 6.2 for Android, replacing
unityLibrary
witharcore_client
,unityandroidpermissions
andUnityARCore
.When using
UnityWidget
in Flutter, setfullscreen: false
to disable fullscreen.
- Open the ios/Runner/Info.plist and change the following:
Setup Vuforia
Thanks to @PiotrxKolasinski for writing down the exact steps:
- Open the android/unityLibrary/build.gradle file and change the following:
- Using Android Studio, go to File > Open and select the android/ folder. A new project will open.
Don't worry if the error message 'Project with path ':VuforiaWrapper' could not be found in project ':unityLibrary' appears. The next step will fix it.
- In this new project window, go to File > New > New Module > Import .JAR/.AAR package and select the android/unityLibrary/libs/VuforiaWrapper.aar file. A new folder named VuforiaWrapper will be created inside android/. You can now close this new project window.
Unity Flutter Widget Github
Communicating #
Flutter-Unity #
On a
UnityWidget
widget, get theUnityWidgetController
received by theonUnityCreated
callback.Use the method
postMessage
to send a string, using the GameObject name and the name of a behaviour method that should be called.
Unity-Flutter #
- Select the GameObject that should execute the communication and go to Inspector > Add Component > Unity Message Manager.
Create a new
MonoBehaviour
subclass and add to the same GameObject as a script.On this new behaviour, call
GetComponent<UnityMessageManager>()
to get aUnityMessageManager
.Use the method
SendMessageToFlutter
to send a string. Receive this message using theonUnityMessage
callback of aUnityWidget
.
Troubleshooting #
Location: Unity
Error:
Solution:
- Open the unity/project-name/Assets/FlutterUnityIntegration/Editor/Build.cs file.
1.1. On line 48, change the following:
1.2. On line 115, change the following:
Location: Android Studio
Error:
Solution:
- Open the android/app/build.gradle file and change the following:
Location: Android Studio
Error:
Solution:
- Open the android/build.gradle file and change the following:
Location: Android Studio
Error:
Solution:
- Open the android/app/build.gradle file and change the following:
Examples #
Simple Example #
Communicating with and from Unity #
Props #
Unity Flutter Download
fullscreen
(Enable or disable fullscreen mode on Android)
API #
pause()
(Use this to pause unity player)resume()
(Use this to resume unity player)unload()
(Use this to unload unity player) *Requires Unity 2019.4.3 or laterquit()
(Use this to quit unity player)postMessage(String gameObject, methodName, message)
(Allows you invoke commands in Unity from flutter)onUnityMessage(data)
(Unity to flutter binding and listener)onUnityUnloaded()
(Unity to flutter listener when unity is unloaded)onUnitySceneLoaded(String name, int buildIndex, bool isLoaded, bool isValid,)
(Unity to flutter binding and listener when new scene is loaded)
Known issues #
Unity Flutter App
- Remember to disabled fullscreen in unity player settings to disable unity fullscreen.
- App crashes on screen exit and re-entry do this
Build Setting - iOS - Other Settings - Configuration - Enable Custom Background Behaviors
Sponsors
Support this project with your organization. Your donations will be used to help children first and then those in need. Your logo will show up here with a link to your website. [Contribute]
Contributors ✨ #
Thanks goes to these wonderful people (emoji key):
Rex Raphael 💻📖💬🐛👀✅ | Thomas Stockx 💻📖💬✅ | Kris Pypen 💻📖💬✅ | Lorant Csonka 📖📹 |
Flutter Unity View Widget
This project follows the all-contributors specification. Contributions of any kind welcome!
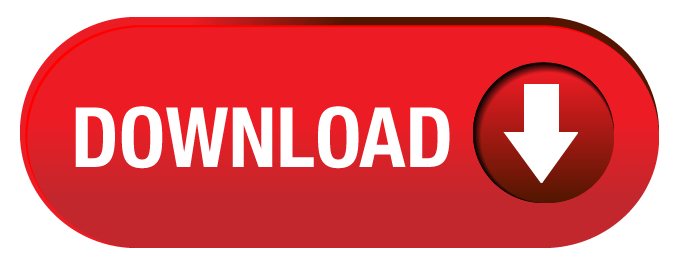